Window
- class Window(*args, **kwargs)
Superclasses: Window
, Widget
, InitiallyUnowned
, Object
Subclasses: AboutWindow
, PreferencesWindow
Implemented Interfaces: Accessible
, Buildable
, ConstraintTarget
, Native
, Root
, ShortcutManager
A freeform window.
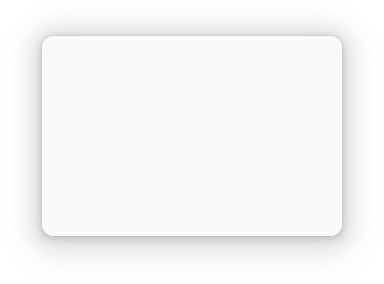
The AdwWindow
widget is a subclass of Window
which has no
titlebar area. Instead, ToolbarView
can be used together with
HeaderBar
or HeaderBar
as follows:
<object class="AdwWindow">
<property name="content">
<object class="AdwToolbarView">
<child type="top">
<object class="AdwHeaderBar"/>
</child>
<property name="content">
<!-- ... -->
</property>
</object>
</property>
</object>
Using titlebar
or child
is not supported and will result in a crash. Use content
instead.
Dialogs
AdwWindow
can contain Dialog
. Use present
with the
window or a widget within a window to show a dialog.
Breakpoints
AdwWindow
can be used with Breakpoint
the same way as
BreakpointBin
. Refer to that widget’s documentation for details.
Example:
<object class="AdwWindow">
<property name="width-request">360</property>
<property name="height-request">200</property>
<property name="content">
<object class="AdwToolbarView">
<child type="top">
<object class="AdwHeaderBar"/>
</child>
<property name="content">
<!-- ... -->
</property>
<child type="bottom">
<object class="GtkActionBar" id="bottom_bar">
<property name="revealed">True</property>
<property name="visible">False</property>
</object>
</child>
</object>
</property>
<child>
<object class="AdwBreakpoint">
<condition>max-width: 500px</condition>
<setter object="bottom_bar" property="visible">True</setter>
</object>
</child>
</object>
Like AdwBreakpointBin
, if breakpoints are used, AdwWindow
doesn’t have a
minimum size, and width_request
and
height_request
properties must be set manually.
Constructors
Methods
- class Window
- add_breakpoint(breakpoint: Breakpoint) None
Adds
breakpoint
toself
.Added in version 1.4.
- Parameters:
breakpoint – the breakpoint to add
- get_content() Widget | None
Gets the content widget of
self
.This method should always be used instead of
get_child
.
- get_current_breakpoint() Breakpoint | None
Gets the current breakpoint.
Added in version 1.4.
- get_dialogs() ListModel
Returns a
ListModel
that contains the open dialogs ofself
.This can be used to keep an up-to-date view.
Added in version 1.5.
Properties
- class Window
-
- props.current_breakpoint: Breakpoint
The current breakpoint.
Added in version 1.4.
Fields
- class Window
- parent_instance