AboutDialog
Added in version 1.5.
Superclasses: Dialog
, Widget
, InitiallyUnowned
, Object
Implemented Interfaces: Accessible
, Buildable
, ConstraintTarget
A dialog showing information about the application.
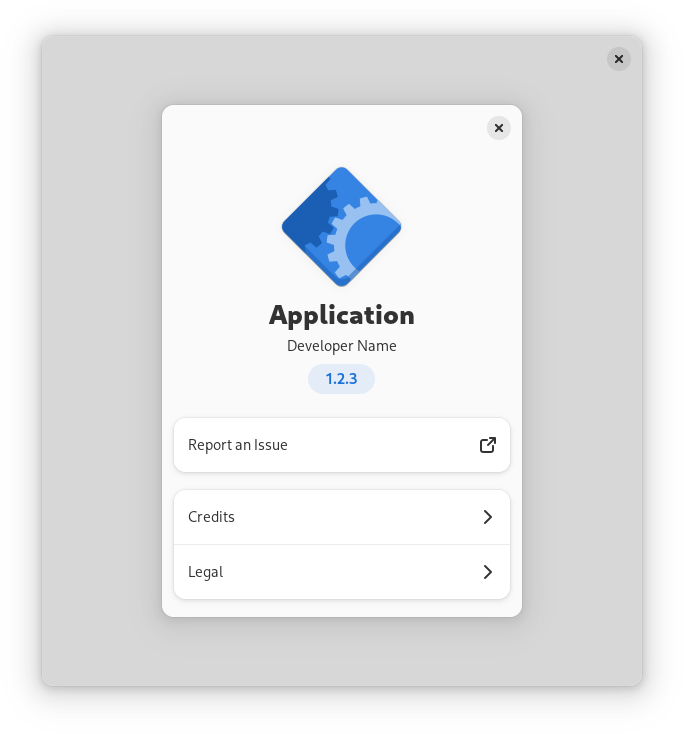
an about dialog is typically opened when the user activates the About …
item in the application’s primary menu. All parts of the dialog are optional.
Main page
AdwAboutDialog
prominently displays the application’s icon, name, developer
name and version. They can be set with the application_icon
,
application_name
,
developer_name
and version
respectively.
What’s New
AdwAboutDialog
provides a way for applications to display their release
notes, set with the release_notes
property.
Release notes are formatted the same way as AppStream descriptions.
The supported formatting options are:
Paragraph (
<p>
)Ordered list (
<ol>
), with list items (<li>
)Unordered list (
<ul>
), with list items (<li>
)
Within paragraphs and list items, emphasis (<em>
) and inline code
(<code>
) text styles are supported. The emphasis is rendered in italic,
while inline code is shown in a monospaced font.
Any text outside paragraphs or list items is ignored.
Nested lists are not supported.
Only one version can be shown at a time. By default, the displayed version
number matches version
. Use
release_notes_version
to override it.
Details
The Details page displays the application comments and links.
The comments can be set with the comments
property.
Unlike comments
, this string can be long and
detailed. It can also contain links and Pango markup.
To set the application website, use website
.
To add extra links below the website, use add_link
.
If the Details page doesn’t have any other content besides website, the website will be displayed on the main page instead.
Troubleshooting
AdwAboutDialog
displays the following two links on the main page:
Support Questions, set with the
support_url
property,Report an Issue, set with the
issue_url
property.
Additionally, applications can provide debugging information. It will be
shown separately on the Troubleshooting page. Use the
debug_info
property to specify it.
It’s intended to be attached to issue reports when reporting issues against the application. As such, it cannot contain markup or links.
AdwAboutDialog
provides a quick way to save debug information to a file.
When saving, debug_info_filename
would be used as
the suggested filename.
Credits and Acknowledgements
The Credits page has the following default sections:
Developers, set with the
developers
property,Designers, set with the
designers
property,Artists, set with the
artists
property,Documenters, set with the
documenters
property,Translators, set with the
translator_credits
property.
When setting translator credits, use the strings "translator-credits"
or
"translator_credits"
and mark them as translatable.
The default sections that don’t contain any names won’t be displayed.
The Credits page can also contain an arbitrary number of extra sections below
the default ones. Use add_credit_section
to add them.
The Acknowledgements page can be used to acknowledge additional people and
organizations for their non-development contributions. Use
add_acknowledgement_section
to add sections to it. For
example, it can be used to list backers in a crowdfunded project or to give
special thanks.
Each of the people or organizations can have an email address or a website
specified. To add a email address, use a string like
Edgar Allan Poe <edgar``poe`
.com>`. To specify a website with a title, use a
string like The GNOME Project https://www.gnome.org
:

Legal
The Legal page displays the copyright and licensing information for the application and other modules.
The copyright string is set with the copyright
property and should be a short string of one or two lines, for example:
© 2022 Example
.
Licensing information can be quickly set from a list of known licenses with
the license_type
property. If the application’s
license is not in the list, license
can be used
instead.
To add information about other modules, such as application dependencies or
data, use add_legal_section
.
Constructing
To make constructing an AdwAboutDialog
as convenient as possible, you can
use the function show_about_dialog
which constructs and shows a
dialog.
static void
show_about (GtkApplication *app)
{
const char *developers[] = {
"Angela Avery",
NULL
};
const char *designers[] = {
"GNOME Design Team",
NULL
};
adw_show_about_dialog (GTK_WIDGET (gtk_application_get_active_window (app)),
"application-name", _("Example"),
"application-icon", "org.example.App",
"version", "1.2.3",
"copyright", "© 2022 Angela Avery",
"issue-url", "https://gitlab.gnome.org/example/example/-/issues/new",
"license-type", GTK_LICENSE_GPL_3_0,
"developers", developers,
"designers", designers,
"translator-credits", _("translator-credits"),
NULL);
}
CSS nodes
AdwAboutDialog
has a main CSS node with the name dialog
and the
style class .about
.
Constructors
- class AboutDialog
-
- classmethod new_from_appdata(resource_path: str, release_notes_version: str | None = None) Dialog
Creates a new
AdwAboutDialog
using AppStream metadata.This automatically sets the following properties with the following AppStream values:
application_icon
is set from the<id>
application_name
is set from the<name>
developer_name
is set from the<name>
within<developer>
version
is set from the version of the latest releasewebsite
is set from the<url type="homepage">
support_url
is set from the<url type="help">
issue_url
is set from the<url type="bugtracker">
license_type
is set from the<project_license>
.If the license type retrieved from AppStream is not listed in
License
, it will be set toGTK_LICENCE_CUSTOM
.
If
release_notes_version
is notNULL
,release_notes_version
is set to match it, whilerelease_notes
is set from the AppStream release description for that version.Added in version 1.5.
- Parameters:
resource_path – The resource to use
release_notes_version – The version to retrieve release notes for
Methods
- class AboutDialog
- add_acknowledgement_section(name: str | None, people: Sequence[str]) None
Adds a section to the Acknowledgements page.
This can be used to acknowledge additional people and organizations for their non-development contributions - for example, backers in a crowdfunded project.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
add_credit_section
Added in version 1.5.
- Parameters:
name – the section name
people – the list of names
- add_credit_section(name: str | None, people: Sequence[str]) None
Adds an extra section to the Credits page.
Extra sections are displayed below the standard categories.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
add_acknowledgement_section
Added in version 1.5.
- Parameters:
name – the section name
people – the list of names
- add_legal_section(title: str, copyright: str | None, license_type: License, license: str | None = None) None
Adds an extra section to the Legal page.
Extra sections will be displayed below the application’s own information.
The parameters
copyright
,license_type
andlicense
will be used to present the it the same way ascopyright
,license_type
andlicense
are for the application’s own information.See those properties for more details.
This can be useful to attribute the application dependencies or data.
Examples:
adw_about_dialog_add_legal_section (ADW_ABOUT_DIALOG (about), _("Copyright and a known license"), "© 2022 Example", GTK_LICENSE_LGPL_2_1, NULL); adw_about_dialog_add_legal_section (ADW_ABOUT_DIALOG (about), _("Copyright and custom license"), "© 2022 Example", GTK_LICENSE_CUSTOM, "Custom license text"); adw_about_dialog_add_legal_section (ADW_ABOUT_DIALOG (about), _("Copyright only"), "© 2022 Example", GTK_LICENSE_UNKNOWN, NULL); adw_about_dialog_add_legal_section (ADW_ABOUT_DIALOG (about), _("Custom license only"), NULL, GTK_LICENSE_CUSTOM, "Something completely custom here.");
Added in version 1.5.
- Parameters:
title – the name of the section
copyright – a copyright string
license_type – the type of license
license – custom license information
- add_link(title: str, url: str) None
Adds an extra link to the Details page.
Extra links are displayed under the comment and website.
Underlines in
title
will be interpreted as indicating a mnemonic.See
website
.Added in version 1.5.
- Parameters:
title – the link title
url – the link URL
- get_designers() list[str] | None
Gets the list of designers of the application.
Added in version 1.5.
- get_developers() list[str] | None
Gets the list of developers of the application.
Added in version 1.5.
- get_documenters() list[str] | None
Gets the list of documenters of the application.
Added in version 1.5.
- get_release_notes_version() str
Gets the version described by the application’s release notes.
Added in version 1.5.
- set_application_icon(application_icon: str) None
Sets the name of the application icon for
self
.The icon is displayed at the top of the main page.
Added in version 1.5.
- Parameters:
application_icon – the application icon name
- set_application_name(application_name: str) None
Sets the application name for
self
.The name is displayed at the top of the main page.
Added in version 1.5.
- Parameters:
application_name – the application name
- set_artists(artists: Sequence[str] | None = None) None
Sets the list of artists of the application.
It will be displayed on the Credits page.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
add_credit_section
add_acknowledgement_section
Added in version 1.5.
- Parameters:
artists – the list of artists
- set_comments(comments: str) None
Sets the comments about the application.
Comments will be shown on the Details page, above links.
Unlike
comments
, this string can be long and detailed. It can also contain links and Pango markup.Added in version 1.5.
- Parameters:
comments – the comments
- set_copyright(copyright: str) None
Sets the copyright information for
self
.This should be a short string of one or two lines, for example:
© 2022 Example
.The copyright information will be displayed on the Legal page, before the application license.
add_legal_section
can be used to add copyright information for the application dependencies or other components.Added in version 1.5.
- Parameters:
copyright – the copyright information
- set_debug_info(debug_info: str) None
Sets the debug information for
self
.Debug information will be shown on the Troubleshooting page. It’s intended to be attached to issue reports when reporting issues against the application.
AdwAboutDialog
provides a quick way to save debug information to a file. When saving,debug_info_filename
would be used as the suggested filename.Debug information cannot contain markup or links.
Added in version 1.5.
- Parameters:
debug_info – the debug information
- set_debug_info_filename(filename: str) None
Sets the debug information filename for
self
.It will be used as the suggested filename when saving debug information to a file.
See
debug_info
.Added in version 1.5.
- Parameters:
filename – the debug info filename
- set_designers(designers: Sequence[str] | None = None) None
Sets the list of designers of the application.
It will be displayed on the Credits page.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
add_credit_section
add_acknowledgement_section
Added in version 1.5.
- Parameters:
designers – the list of designers
- set_developer_name(developer_name: str) None
Sets the developer name for
self
.The developer name is displayed on the main page, under the application name.
If the application is developed by multiple people, the developer name can be set to values like “AppName team”, “AppName developers” or “The AppName project”, and the individual contributors can be listed on the Credits page, with
developers
and related properties.Added in version 1.5.
- Parameters:
developer_name – the developer name
- set_developers(developers: Sequence[str] | None = None) None
Sets the list of developers of the application.
It will be displayed on the Credits page.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
add_credit_section
add_acknowledgement_section
Added in version 1.5.
- Parameters:
developers – the list of developers
- set_documenters(documenters: Sequence[str] | None = None) None
Sets the list of documenters of the application.
It will be displayed on the Credits page.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
add_credit_section
add_acknowledgement_section
Added in version 1.5.
- Parameters:
documenters – the list of documenters
- set_issue_url(issue_url: str) None
Sets the issue tracker URL for
self
.The issue tracker link is displayed on the main page.
Added in version 1.5.
- Parameters:
issue_url – the issue tracker URL
- set_license(license: str) None
Sets the license for
self
.This can be used to set a custom text for the license if it can’t be set via
license_type
.When set,
license_type
will be set toGTK_LICENSE_CUSTOM
.The license text will be displayed on the Legal page, below the copyright information.
License text can contain Pango markup and links.
add_legal_section
can be used to add license information for the application dependencies or other components.Added in version 1.5.
- Parameters:
license – the license
- set_license_type(license_type: License) None
Sets the license for
self
from a list of known licenses.If the application’s license is not in the list,
license
can be used instead. The license type will be automatically set toGTK_LICENSE_CUSTOM
in that case.If
license_type
isGTK_LICENSE_UNKNOWN
, no information will be displayed.If
license_type
is different fromGTK_LICENSE_CUSTOM
.license
will be cleared out.The license description will be displayed on the Legal page, below the copyright information.
add_legal_section
can be used to add license information for the application dependencies or other components.Added in version 1.5.
- Parameters:
license_type – the license type
- set_release_notes(release_notes: str) None
Sets the release notes for
self
.Release notes are displayed on the the What’s New page.
Release notes are formatted the same way as AppStream descriptions.
The supported formatting options are:
Paragraph (
<p>
)Ordered list (
<ol>
), with list items (<li>
)Unordered list (
<ul>
), with list items (<li>
)
Within paragraphs and list items, emphasis (
<em>
) and inline code (<code>
) text styles are supported. The emphasis is rendered in italic, while inline code is shown in a monospaced font.Any text outside paragraphs or list items is ignored.
Nested lists are not supported.
AdwAboutDialog
displays the version above the release notes. If set, therelease_notes_version
of the property will be used as the version; otherwise,version
is used.Added in version 1.5.
- Parameters:
release_notes – the release notes
- set_release_notes_version(version: str) None
Sets the version described by the application’s release notes.
The release notes version is displayed on the What’s New page, above the release notes.
If not set,
version
will be used instead.For example, an application with the current version 2.0.2 might want to keep the release notes from 2.0.0, and set the release notes version accordingly.
See
release_notes
.Added in version 1.5.
- Parameters:
version – the release notes version
- set_support_url(support_url: str) None
Sets the URL of the support page for
self
.The support page link is displayed on the main page.
Added in version 1.5.
- Parameters:
support_url – the support page URL
- set_translator_credits(translator_credits: str) None
Sets the translator credits string.
It will be displayed on the Credits page.
This string should be
"translator-credits"
or"translator_credits"
and should be marked as translatable.The string may contain email addresses and URLs, see the introduction for more details.
See also:
add_credit_section
add_acknowledgement_section
Added in version 1.5.
- Parameters:
translator_credits – the translator credits
- set_version(version: str) None
Sets the version for
self
.The version is displayed on the main page.
If
release_notes_version
is not set, the version will also be displayed above the release notes on the What’s New page.Added in version 1.5.
- Parameters:
version – the version
- set_website(website: str) None
Sets the application website URL for
self
.Website is displayed on the Details page, below comments, or on the main page if the Details page doesn’t have any other content.
Applications can add other links below, see
add_link
.Added in version 1.5.
- Parameters:
website – the website URL
Properties
- class AboutDialog
- props.application_icon: str
The name of the application icon.
The icon is displayed at the top of the main page.
Added in version 1.5.
- props.application_name: str
The name of the application.
The name is displayed at the top of the main page.
Added in version 1.5.
- props.artists: Sequence[str]
The list of artists of the application.
It will be displayed on the Credits page.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
add_credit_section
add_acknowledgement_section
Added in version 1.5.
- props.comments: str
The comments about the application.
Comments will be shown on the Details page, above links.
Unlike
comments
, this string can be long and detailed. It can also contain links and Pango markup.Added in version 1.5.
- props.copyright: str
The copyright information.
This should be a short string of one or two lines, for example:
© 2022 Example
.The copyright information will be displayed on the Legal page, above the application license.
add_legal_section
can be used to add copyright information for the application dependencies or other components.Added in version 1.5.
- props.debug_info: str
The debug information.
Debug information will be shown on the Troubleshooting page. It’s intended to be attached to issue reports when reporting issues against the application.
AdwAboutDialog
provides a quick way to save debug information to a file. When saving,debug_info_filename
would be used as the suggested filename.Debug information cannot contain markup or links.
Added in version 1.5.
- props.debug_info_filename: str
The debug information filename.
It will be used as the suggested filename when saving debug information to a file.
See
debug_info
.Added in version 1.5.
- props.designers: Sequence[str]
The list of designers of the application.
It will be displayed on the Credits page.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
add_credit_section
add_acknowledgement_section
Added in version 1.5.
- props.developer_name: str
The developer name.
The developer name is displayed on the main page, under the application name.
If the application is developed by multiple people, the developer name can be set to values like “AppName team”, “AppName developers” or “The AppName project”, and the individual contributors can be listed on the Credits page, with
developers
and related properties.Added in version 1.5.
- props.developers: Sequence[str]
The list of developers of the application.
It will be displayed on the Credits page.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
add_credit_section
add_acknowledgement_section
Added in version 1.5.
- props.documenters: Sequence[str]
The list of documenters of the application.
It will be displayed on the Credits page.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
add_credit_section
add_acknowledgement_section
Added in version 1.5.
- props.issue_url: str
The URL for the application’s issue tracker.
The issue tracker link is displayed on the main page.
Added in version 1.5.
- props.license: str
The license text.
This can be used to set a custom text for the license if it can’t be set via
license_type
.When set,
license_type
will be set toGTK_LICENSE_CUSTOM
.The license text will be displayed on the Legal page, below the copyright information.
License text can contain Pango markup and links.
add_legal_section
can be used to add license information for the application dependencies or other components.Added in version 1.5.
- props.license_type: License
The license type.
Allows to set the application’s license froma list of known licenses.
If the application’s license is not in the list,
license
can be used instead. The license type will be automatically set toGTK_LICENSE_CUSTOM
in that case.If set to
GTK_LICENSE_UNKNOWN
, no information will be displayed.If the license type is different from
GTK_LICENSE_CUSTOM
.license
will be cleared out.The license description will be displayed on the Legal page, below the copyright information.
add_legal_section
can be used to add license information for the application dependencies or other components.Added in version 1.5.
- props.release_notes: str
The release notes of the application.
Release notes are displayed on the the What’s New page.
Release notes are formatted the same way as AppStream descriptions.
The supported formatting options are:
Paragraph (
<p>
)Ordered list (
<ol>
), with list items (<li>
)Unordered list (
<ul>
), with list items (<li>
)
Within paragraphs and list items, emphasis (
<em>
) and inline code (<code>
) text styles are supported. The emphasis is rendered in italic, while inline code is shown in a monospaced font.Any text outside paragraphs or list items is ignored.
Nested lists are not supported.
AdwAboutDialog
displays the version above the release notes. If set, therelease_notes_version
of the property will be used as the version; otherwise,version
is used.Added in version 1.5.
- props.release_notes_version: str
The version described by the application’s release notes.
The release notes version is displayed on the What’s New page, above the release notes.
If not set,
version
will be used instead.For example, an application with the current version 2.0.2 might want to keep the release notes from 2.0.0, and set the release notes version accordingly.
See
release_notes
.Added in version 1.5.
- props.support_url: str
The URL of the application’s support page.
The support page link is displayed on the main page.
Added in version 1.5.
- props.translator_credits: str
The translator credits string.
It will be displayed on the Credits page.
This string should be
"translator-credits"
or"translator_credits"
and should be marked as translatable.The string may contain email addresses and URLs, see the introduction for more details.
See also:
add_credit_section
add_acknowledgement_section
Added in version 1.5.
- props.version: str
The version of the application.
The version is displayed on the main page.
If
release_notes_version
is not set, the version will also be displayed above the release notes on the What’s New page.Added in version 1.5.