Toast
Superclasses: Object
A helper object for ToastOverlay
.
Toasts are meant to be passed into add_toast
as
follows:
adw_toast_overlay_add_toast (overlay, adw_toast_new (_("Simple Toast")));
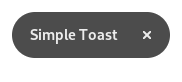
Toasts always have a close button. They emit the dismissed
signal when disappearing.
timeout
determines how long the toast stays on screen, while
priority
determines how it behaves if another toast is
already being displayed.
Toast titles use Pango markup by default, set use_markup
to
FALSE
if this is unwanted.
custom_title
can be used to replace the title label with a
custom widget.
Actions
Toasts can have one button on them, with a label and an attached
Action
.
AdwToast *toast = adw_toast_new (_("Toast with Action"));
adw_toast_set_button_label (toast, _("_Example"));
adw_toast_set_action_name (toast, "win.example");
adw_toast_overlay_add_toast (overlay, toast);
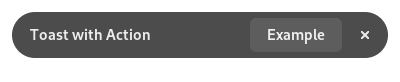
Modifying toasts
Toasts can be modified after they have been shown. For this, an AdwToast
reference must be kept around while the toast is visible.
A common use case for this is using toasts as undo prompts that stack with each other, allowing to batch undo the last deleted items:
static void
toast_undo_cb (GtkWidget *sender,
const char *action,
GVariant *param)
{
// Undo the deletion
}
static void
dismissed_cb (MyWindow *self)
{
self->undo_toast = NULL;
// Permanently delete the items
}
static void
delete_item (MyWindow *self,
MyItem *item)
{
g_autofree char *title = NULL;
int n_items;
// Mark the item as waiting for deletion
n_items = ... // The number of waiting items
if (!self->undo_toast) {
self->undo_toast = adw_toast_new_format (_("‘``%s``’ deleted"), ...);
adw_toast_set_priority (self->undo_toast, ADW_TOAST_PRIORITY_HIGH);
adw_toast_set_button_label (self->undo_toast, _("_Undo"));
adw_toast_set_action_name (self->undo_toast, "toast.undo");
g_signal_connect_swapped (self->undo_toast, "dismissed",
G_CALLBACK (dismissed_cb), self);
adw_toast_overlay_add_toast (self->toast_overlay, self->undo_toast);
return;
}
title =
g_strdup_printf (ngettext ("<span font_features='tnum=1'>``%d``</span> item deleted",
"<span font_features='tnum=1'>``%d``</span> items deleted",
n_items), n_items);
adw_toast_set_title (self->undo_toast, title);
// Bump the toast timeout
adw_toast_overlay_add_toast (self->toast_overlay, g_object_ref (self->undo_toast));
}
static void
my_window_class_init (MyWindowClass *klass)
{
GtkWidgetClass *widget_class = GTK_WIDGET_CLASS (klass);
gtk_widget_class_install_action (widget_class, "toast.undo", NULL, toast_undo_cb);
}
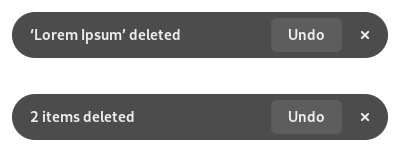
Constructors
Methods
- class Toast
- dismiss() None
Dismisses
self
.Does nothing if
self
has already been dismissed, or hasn’t been added to anToastOverlay
.
- get_priority() ToastPriority
Gets priority for
self
.
- get_title() str | None
Gets the title that will be displayed on the toast.
If a custom title has been set with
set_custom_title
the return value will beNone
.
- set_action_name(action_name: str | None = None) None
Sets the name of the associated action.
It will be activated when clicking the button.
See
action_target
.- Parameters:
action_name – the action name
- set_action_target_value(action_target: Variant | None = None) None
Sets the parameter for action invocations.
If the
action_target
variant has a floating reference this function will sink it.- Parameters:
action_target – the action target
- set_button_label(button_label: str | None = None) None
Sets the label to show on the button.
Underlines in the button text can be used to indicate a mnemonic.
If set to
NULL
, the button won’t be shown.See
action_name
.- Parameters:
button_label – a button label
- set_custom_title(widget: Widget | None = None) None
Sets the custom title widget of
self
.It will be displayed instead of the title if set. In this case,
title
is ignored.Setting a custom title will unset
title
.Added in version 1.2.
- Parameters:
widget – the custom title widget
- set_detailed_action_name(detailed_action_name: str | None = None) None
Sets the action name and its parameter.
detailed_action_name
is a string in the format accepted byparse_detailed_name
.- Parameters:
detailed_action_name – the detailed action name
- set_priority(priority: ToastPriority) None
Sets priority for
self
.Priority controls how the toast behaves when another toast is already being displayed.
If
priority
isADW_TOAST_PRIORITY_NORMAL
, the toast will be queued.If
priority
isADW_TOAST_PRIORITY_HIGH
, the toast will be displayed immediately, pushing the previous toast into the queue instead.- Parameters:
priority – the priority
- set_timeout(timeout: int) None
Sets timeout for
self
.If
timeout
is 0, the toast is displayed indefinitely until manually dismissed.Toasts cannot disappear while being hovered, pressed (on touchscreen), or have keyboard focus inside them.
- Parameters:
timeout – the timeout
- set_title(title: str) None
Sets the title that will be displayed on the toast.
The title can be marked up with the Pango text markup language.
Setting a title will unset
custom_title
.If
custom_title
is set, it will be used instead.- Parameters:
title – a title
- set_use_markup(use_markup: bool) None
Whether to use Pango markup for the toast title.
See also
parse_markup
.Added in version 1.4.
- Parameters:
use_markup – whether to use markup
Properties
- class Toast
- props.action_name: str
The name of the associated action.
It will be activated when clicking the button.
See
action_target
.
- props.button_label: str
The label to show on the button.
Underlines in the button text can be used to indicate a mnemonic.
If set to
NULL
, the button won’t be shown.See
action_name
.
- props.custom_title: Widget
The custom title widget.
It will be displayed instead of the title if set. In this case,
title
is ignored.Setting a custom title will unset
title
.Added in version 1.2.
- props.priority: ToastPriority
The priority of the toast.
Priority controls how the toast behaves when another toast is already being displayed.
If the priority is
ADW_TOAST_PRIORITY_NORMAL
, the toast will be queued.If the priority is
ADW_TOAST_PRIORITY_HIGH
, the toast will be displayed immediately, pushing the previous toast into the queue instead.
- props.timeout: int
The timeout of the toast, in seconds.
If timeout is 0, the toast is displayed indefinitely until manually dismissed.
Toasts cannot disappear while being hovered, pressed (on touchscreen), or have keyboard focus inside them.
- props.title: str
The title of the toast.
The title can be marked up with the Pango text markup language.
Setting a title will unset
custom_title
.If
custom_title
is set, it will be used instead.
- props.use_markup: bool
Whether to use Pango markup for the toast title.
See also
parse_markup
.Added in version 1.4.